Introduction to Gooey
Gooey is a lightweight, cross-platform GUI framework for building native-feeling applications with a single codebase. Written in pure C, Gooey provides a simple yet powerful API for creating beautiful user interfaces.
Note: Web support is still in development, will release on v1.0.3.
Customize your build of Gooey
If you're building from source you can enable/disable features in include/user_config.h
.
#ifndef USER_CONFIG_H
#define USER_CONFIG_H
// DEBUG/PROD
#define PROJECT_BRANCH 1 // 0=debug 1=prod TODO
// MAX
#define MAX_TIMERS 100 /** Maximum number of timer objects. */
#define MAX_WIDGETS 100 /** Maximum number of widgets that can be added to a window. */
#define MAX_MENU_CHILDREN 10 /** Maximum number of menu children. */
#define MAX_RADIO_BUTTONS 10 /** Maximum number of radio buttons in a group. */
#define MAX_PLOT_COUNT 100 /** Maximum number of plots. */
#define MAX_TABS 50 /** Maximum number of tabs. */
// ENABLE/DISABLE WIDGETS
#define ENABLE_BUTTON 1
#define ENABLE_CANVAS 1
#define ENABLE_CHECKBOX 1
#define ENABLE_CTX_MENU 1 // TODO
#define ENABLE_DEBUG_OVERLAY 1
#define ENABLE_DROP_SURFACE 1
#define ENABLE_DROPDOWN 1
#define ENABLE_IMAGE 1
#define ENABLE_LABEL 1
#define ENABLE_LAYOUT 1
#define ENABLE_LIST 1
#define ENABLE_MENU 1
#define ENABLE_METER 1
#define ENABLE_PLOT 1
#define ENABLE_PROGRESSBAR 1
#define ENABLE_RADIOBUTTON 1
#define ENABLE_SLIDER 1
#define ENABLE_TABS 1
#define ENABLE_TEXTBOX 1
#endif
Installation
To get started with Gooey, download the latest release for your platform:
Linux
# For X11 systems:
wget https://github.com/GooeyUI/GooeyGUI/releases/download/v1.0.2/gooey_x11.tar.gz
# Extract archive
tar -xvzf gooey_x11.tar.gz
# move .so lib files into /usr/local/lib/
Windows
# Download the dlls from:
# https://github.com/GooeyUI/GooeyGUI/releases/download/v1.0.2/gooey_win32.zip
Note: For older releases visit the release page.
Quickstart
Here's a simple hello world example with a message box widget:
#include "gooey.h"
#include <stdio.h>
#include <stdbool.h>
bool state = false;
GooeyWindow *msgBox;
void messageBoxCallback(int option)
{
LOG_INFO("MessageBox option: %d", option);
}
void onButtonClick()
{
state = !state;
GooeyWindow_MakeVisible(msgBox, state);
}
int main()
{
Gooey_Init();
GooeyWindow* win = GooeyWindow_Create("Hello World", 400, 300, true);
msgBox = GooeyMessageBox_Create("Hello", "Welcome to Gooey!", MSGBOX_INFO, messageBoxCallback);
GooeyMessageBox_Show(&msgBox);
GooeyButton *btn = GooeyButton_Create("Click Me", 150, 100, 100, 40, onButtonClick);
GooeyWindow_Run(2, win, msgBox);
GooeyWindow_Cleanup(2, win, msgBox);
return 0;
}
Important: Remember to link against the Gooey library when compiling -lGooeyGUI
and to provide the installation .so or .dll folder -Lfolder_containing_lib
Searching for full API reference? visit here
Architecture
Gooey follows a simple yet powerful architecture designed for performance and flexibility:
Component Hierarchy
The Gooey framework is built around these core components:
- Application - The main container that manages windows and global state
- Window - Top-level container that holds widgets and handles platform-specific rendering
- Widgets - UI elements like buttons, text inputs, and containers
- Canvas - Drawing surface for custom rendering
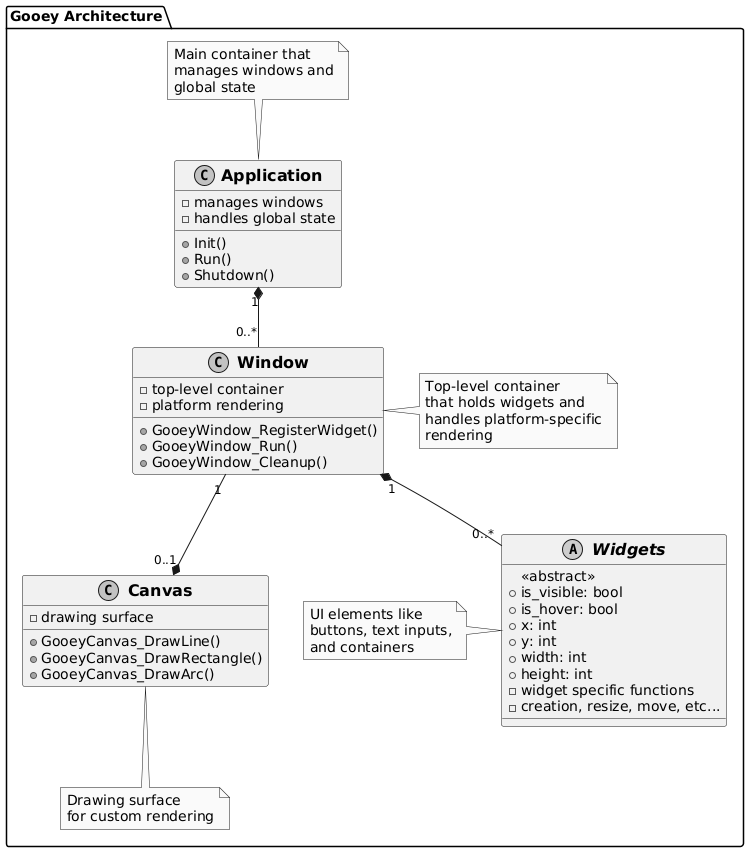
Widgets
Widgets are the building blocks of Gooey applications. All widgets share common properties and methods:
Common Widget Properties
Property | Type | Description |
---|---|---|
is_visible | bool | Controls widget visibility |
type | WIDGET_TYPE | Contains the widget type |
x | int | x coordinate. |
y | int | y coordinate. |
width | int | Width of widget. |
height | int | Height of widget |
Widget Types
Gooey provides several built-in widget types:
- Basic Controls: Button, Label, Checkbox, RadioButton, etc...
- Text Input: Textbox
- Containers: Layout, Tab, etc...
- Specialized: ProgressBar, Slider, Meter, etc...
// Example widget creation
GooeyButton* btn = GooeyButton_Create("Click Me", 10, 10, 100, 40);
GooeyLabel* lbl = GooeyLabel_Create("Hello world", 0.26f, 50, 50);
// Add to window
GooeyWindow_RegisterWidget(window, (void*)btn);
GooeyWindow_RegisterWidget(window, (void*)lbl);
Layout System
Gooey offers flexible layout options for responsive UI design:
Layout
Use layouts for responsive designs:
// Create a vertical layout
GooeyLayout* layout = GooeyLayout_Create(LAYOUT_VERTICAL, 10, 10, 400, 400);
// Add widget
GooeyLayout_AddChild(layout, widget);
// Build layout
GooeyLayout_Build(layout);
Alignment
Control widget positioning within their allocated space:
Property | Type | Description |
---|---|---|
Grid Layout | LAYOUT_GRID | Put your widgets in a grid layout. |
Vertical Layout | LAYOUT_VERTICAL | Put your widgets in a vertical layout. |
Horizontal Layout | LAYOUT_HORIZONTAL | Put your widgets in a horizontal layout. |